Introduction
Laravel is a renowned PHP web-based framework that has earned widespread recognition in web development because of its beautiful syntax, robust features, and effective performance. In this complete tutorial on Laravel development, we’ll examine the key aspects of Laravel, including its architecture and development basics to the most advanced methods and the best methods.
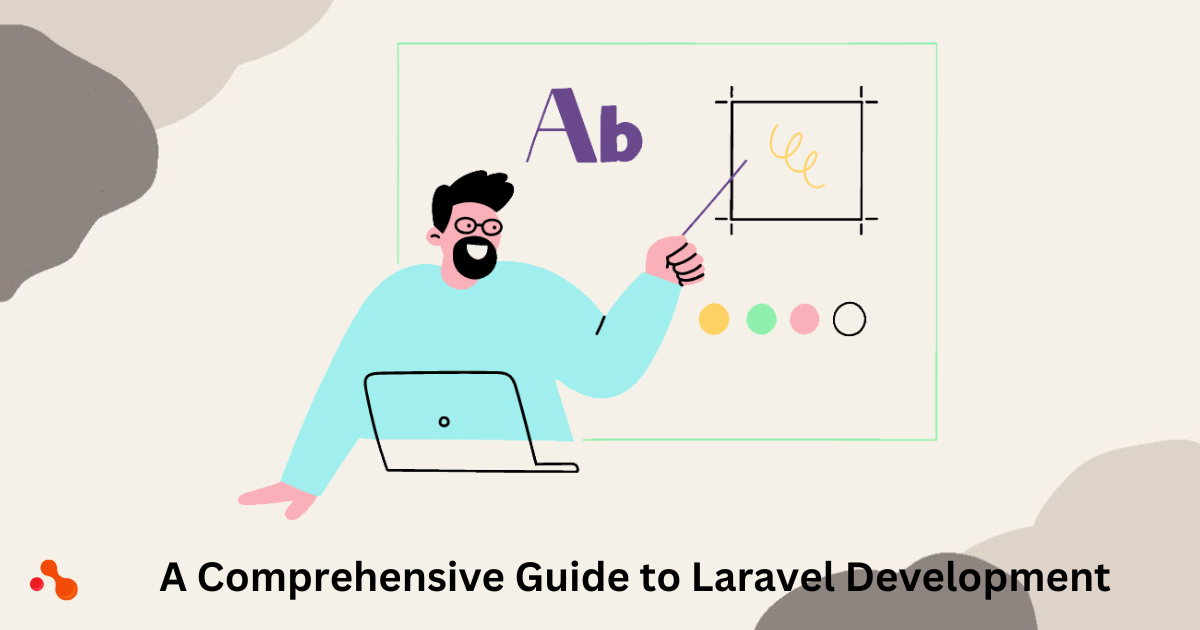
Laravel is gaining popularity because of its simplicity and user-friendliness, which makes it a great choice for web developers seeking to develop complex web-based applications. This article will give an overview of Laravel’s structure and key features. We will also discuss the latest Laravel development methods, the most effective practices, safety issues, and strategies to optimize the performance of your application.
At the end of this article, you’ll be able to comprehend the basics of Laravel and have the skills to create your Laravel applications.
What is Laravel?
Laravel is an open-source PHP web application framework for creating web applications following the Model-View-Controller (MVC) architectural pattern. It was developed by Taylor Otwell in 2011 and has seen significant growth in popularity among developers due to its evocative syntax, beautiful design, and powerful capabilities.
The primary goal of Laravel is to ease web development by offering an extensive set of options and tools that help developers create applications swiftly and effectively. Laravel lets developers concentrate on the core of their application, handling routine tasks that are repetitive and tedious such as Authentication as well as routing, caching as well as session administration.
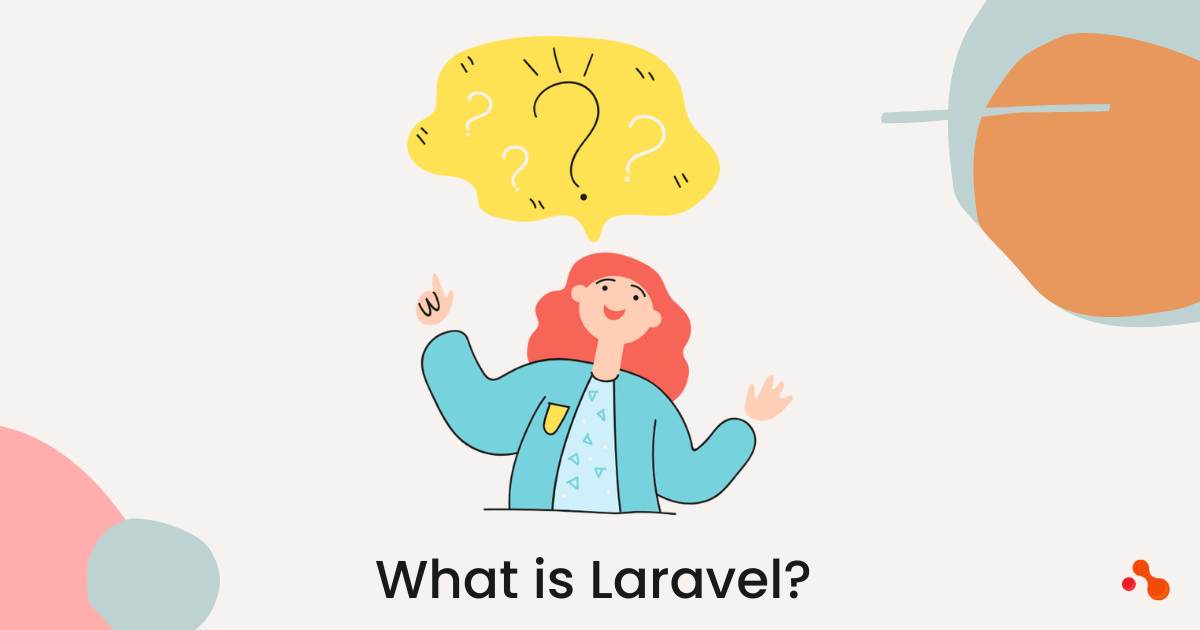
The most important aspects of Laravel include the following:
- Artisan commands-line interface Laravel has a command line-based interface called Artisan that allows users to do routine tasks in development, such as migrating databases, creating boilerplate code, and running tests.
- Blade templating engine Laravel has a powerful and lightweight engine called Blade that allows developers to build designs and templates reused in their web-based applications.
- Eloquent ORM: Laravel has an intuitive and user-friendly Object-Relational Mapping (ORM) system known as Eloquent, which allows developers to communicate with their databases with a simple and elegant syntax.
- Routes: Laravel offers a straightforward and powerful routing system that allows developers to design clear and understandable URLs for their web-based applications.
Compared with other PHP frameworks like CodeIgniter, Symfony, and Yii, Laravel stands out because of its beautiful syntax, powerful features, and slick ORM system. Laravel is also renowned for its extensive and supportive community that offers excellent tutorials, documentation, and tools for developers to study and improve their abilities.
If you’re searching for Laravel solutions for your development needs, you can hire remote developer or consult a Laravel web development firm. A dependable Laravel development firm will help you create custom web applications tailored to your business’s needs, giving you an advantage in the market.
Setting Up Laravel Development Environment
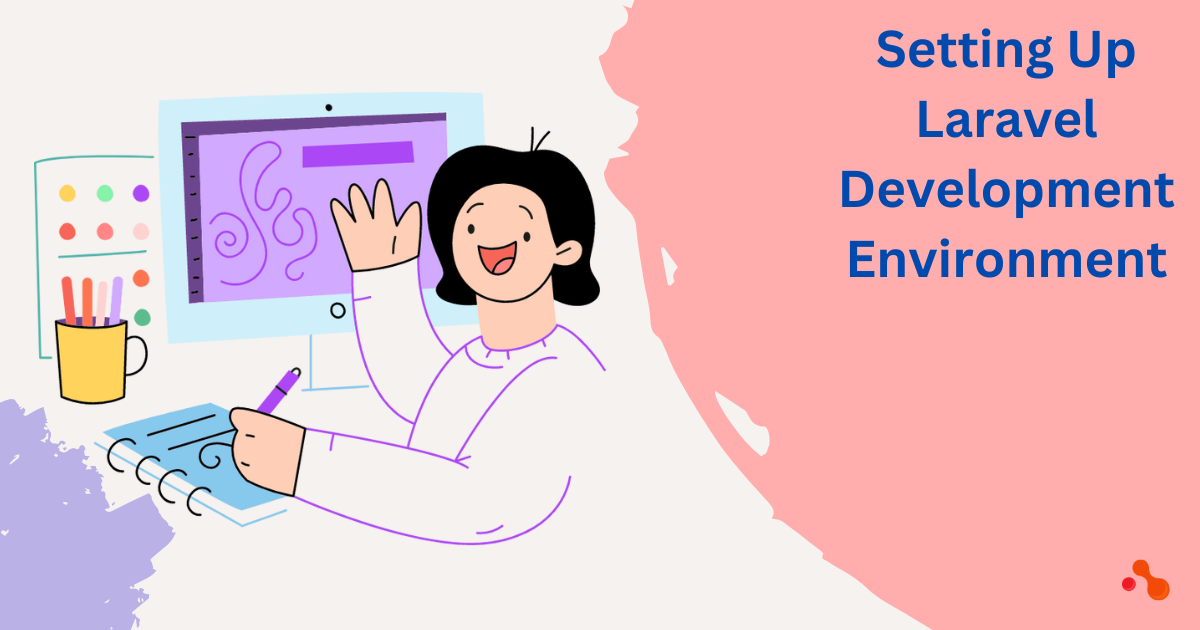
To get started creating with Laravel, it is necessary to have a certain program installed on your PC. The system needs to be required for Laravel development:
- PHP (version 7.3 or greater)
- Composer (a dependency manager for PHP)
- Web servers (such as Apache as well as Nginx)
- Database (such as MySQL or PostgreSQL)
After installing the software, it is time to set up the Laravel developing environment. These are the fundamental steps:
- Install Composer: Download and install Composer from https://getcomposer.org/download/. Follow the installation guideline for your operating system.
- Create a brand new Laravel project Start by opening the terminal, or command prompt and navigate to the directory from which you’d like to build the Laravel project. The following command should be executed:
luaCopy code
composer create-project –prefer-dist laravel/laravel project
The process will result in the creation of a brand new Laravel project placed in the “my project” directory.
- Set up your web server: Laravel comes with an integrated development server. However, you need to configure your web server for production environments. Follow the directions for the specific server you must configure to be compatible with the Laravel project.
- Configure your database. Open your .env file within the Laravel project and update the database settings to match the locally developed environment. If, for instance, you’re using MySQL, the settings could be like this:
MakefileCopy code
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=mydatabase
DB_USERNAME=root
DB_PASSWORD=
That’s it! You’ve got a Laravel Development environment. You are in the process of building your first web-based application.
Laravel Development Fundamentals
Laravel is a PHP framework that allows developers to create web-based applications quickly and effortlessly. In this article, we will go over the basics of Laravel development.
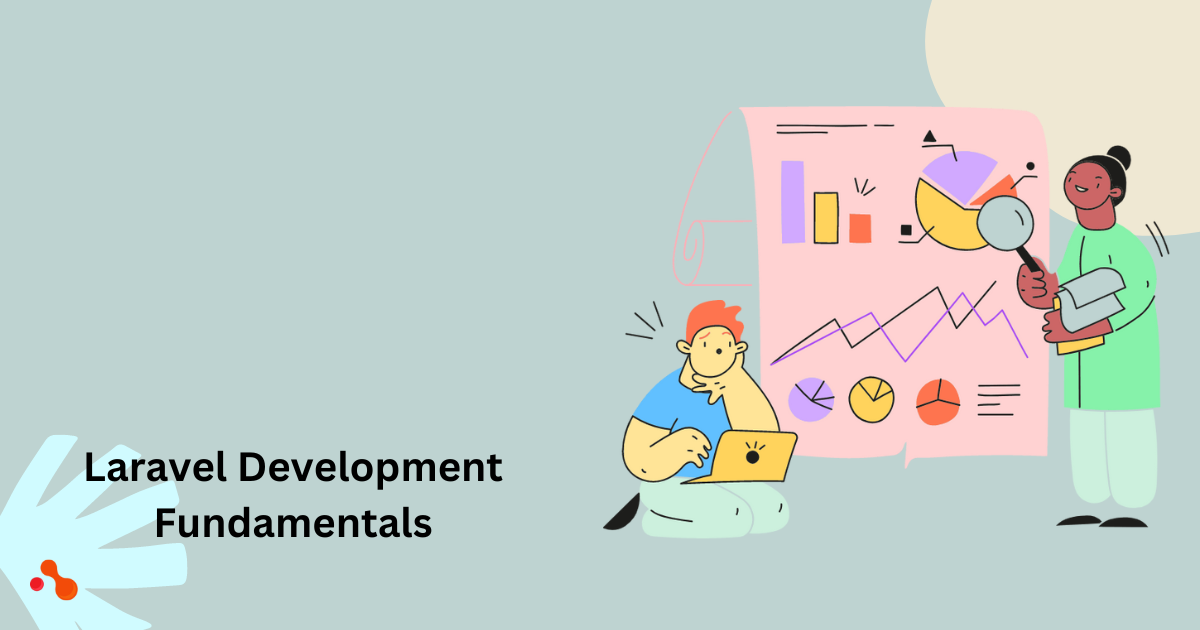
The creation of a new Laravel project
To create a brand new Laravel Project, you need to install Laravel on your PC initially. After that, you can use the Laravel command-line interface (CLI) to create an entirely new project. Here’s how:
- Start your terminal or open a command prompt.
- Go to the directory in which you’d like to start the project. Navigate to the directory in which you would like to create the.
- The following command should be run:
arduinoCopy code
laravel new project
This will result in a new Laravel “project” in the current directory.
Setting up the database
Laravel uses an internal database to store and retrieve information for your web application. Modifying the .env file within the Laravel project is necessary to set up the database. Here’s an example of how the .env file could appear:
MakefileCopy code
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=mydatabase
DB_USERNAME=myusername
DB_PASSWORD=mypassword
This configuration file instructs Laravel to make use of the MySQL database that is running on a local host, with the database named “mydatabase” and the username and password “myusername” and “mypassword,” respectively.
Management of routes
Routes determine the URLs your web application responds to. Laravel offers an easy way to define routes by using your web.php file. Here’s a sample:
sqlCopy code
The Route is: get (‘/,’ function () {
Return view(‘welcome’);
});
This Route instructs Laravel to reply to the URL (“/”) by sending a view titled “welcome.” Creating complex routes that can accept parameters, utilize middleware, and much more is possible.
Example application
Let’s make a basic web application that shows the book’s list of contents to illustrate these ideas. These are the steps:
- Create the new Laravel project by using the CLI.
- Create the database in the .env file.
- Create a brand new database table known as “books” with columns for “id,” “title,” and “author.”
- Create the new model “Book” using the CLI.
- Create a brand new controller named “BookController” using the CLI.
- You can define a route within web.php. Create a route in the web.php file corresponding to a method within the BookController.
- In the BookController approach, take the list of books from the database, and pass it to the view.
- Create a new view called “books.blade.php” that displays the book list.
This simple application shows how Laravel can develop an application for the web quickly and effortlessly.
Advanced Laravel Development Techniques
After you’ve learned the fundamentals of Laravel development, you’ll be able to discover more advanced techniques to elevate your web development projects to the next step. Three advanced Laravel development methods you need to be aware of:
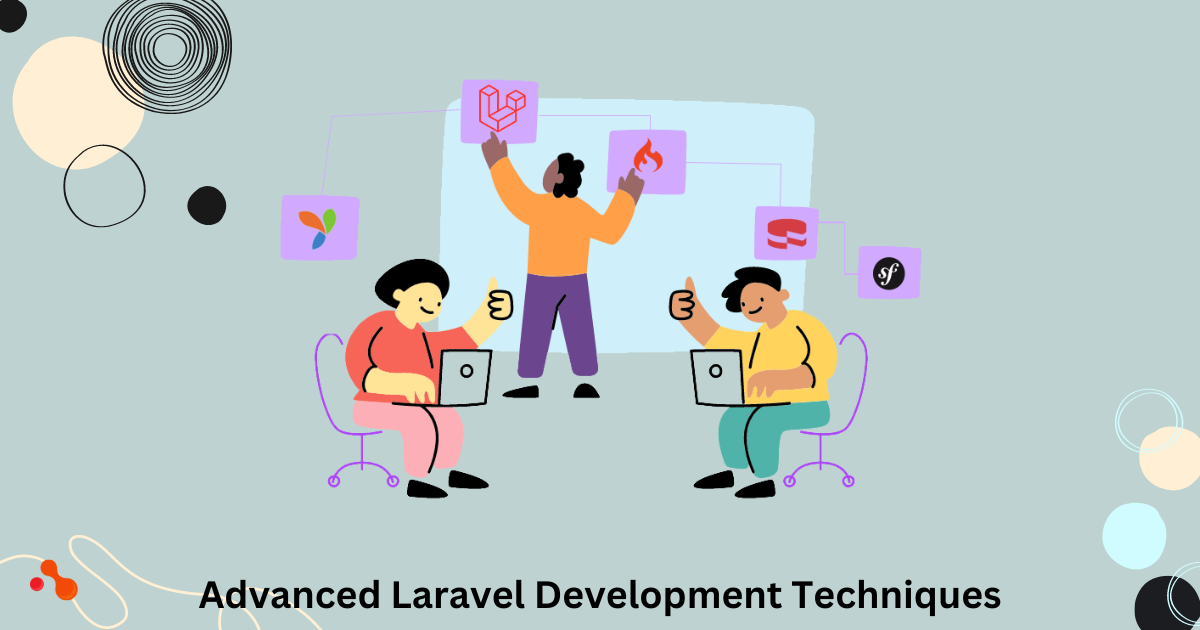
- Authorization and Authentication: Authorization authenticates a user’s identity, while authorization permits access to certain resources depending on the user’s role and authorizations. Laravel lets you create Authentication and Authorization using built-in features, such as security guards and policies. For instance, you could create a login page that authenticates the user against a table in a database like this:
PHPCopy code
public function login(Request $request)
{
$credentials = $request->only(’email’, ‘password’);
if (Auth::attempt($credentials)) {
//Authentication passed…
return redirect()->intended(‘dashboard’);
}
return redirect()->back()->withErrors([
“email” => The credentials provided are not in our database. ‘,
]);
}
- Caching: Caching stores frequently used data in memory to facilitate faster access. Laravel provides a straightforward caching API, which supports a variety of caching drivers, such as database, file, and Redis. For instance, you could store the results of a query in a database like this:
Code for cssCopy
$users = Cache::remember(‘users’, $minutes, function () {
return DB::table(‘users’)->get();
});
- Queues: Queues let you transfer the time-consuming task to background processes for better performance and scaling. Laravel supports several queue drivers, including Beanstalkd, Redis, and Amazon SQS. For instance, you could create a job to send an email to users in the following manner:
PHPCopy code
Public Function Handle()
{
$user = User::find($this->userId);
Mail::to($user->email)->send(new OrderShipped($order));
}
When you master these advanced Laravel development methods, You can build more secure, flexible, and effective web applications.
Laravel Development Best Practices
Laravel development comes with a variety of best practices that you must use to ensure that your code is efficient, secure, and optimized for performance. Here are some helpful tips and examples to follow the best practice guidelines:
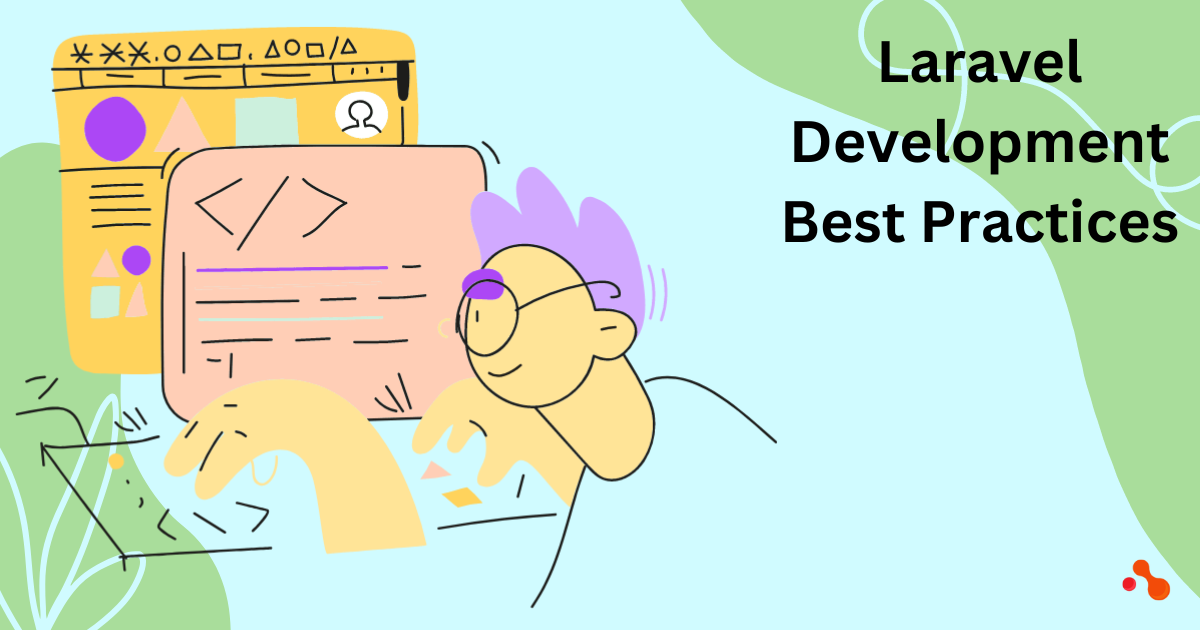
- Code organization: Use your code’s organization guidelines: Follow the PSR-4 standard for class and file naming and grouping related files in folders, and use namespaces to avoid naming conflicts. As an example, you can arrange your Laravel project this way:
markdownCopy code
app/
Http/
Controllers/
HomeController.php
Models/
User.php
Providers/
AppServiceProvider.php
- Security tips: Use Laravel’s security features built into the platform, like verification, CSRF protection, and hashing passwords. Beware of storing sensitive information with plaintext or using insecure encryption algorithms. For instance, you can utilize Laravel’s validation features in the following manner:
bashCopy code
$request->validate([
‘name’ => ‘required|string|max:255′,
’email’ => ‘required|string|email|max:255|unique:users’,
‘password’ => ‘required|string|min:8|confirmed’,
]);
- Performance optimization: Use the caching feature, lazy loading, and other techniques to optimize performance to boost the speed at which you run your Laravel application’s performance. For instance, you can make use of Laravel’s caching function like this:
CssCopy code
$users = Cache::remember(‘users’, 60, function () {
return DB::table(‘users’)->get();
});
Suppose you follow these guidelines to improve the reliability and quality for quality of your Laravel code.
Conclusion
In short, Laravel is a popular PHP framework that makes it easier to manage web development and provides various options to make your website reliable, scalable, and effective. Using Laravel reduces development time and costs while still delivering high-quality web apps.
The post What is Laravel? A Comprehensive Guide to Laravel Development appeared first on Datafloq.